刷题之旅从数组类型的题目开始。第九道题目是杨辉三角2,对应leetcode的题号为119。
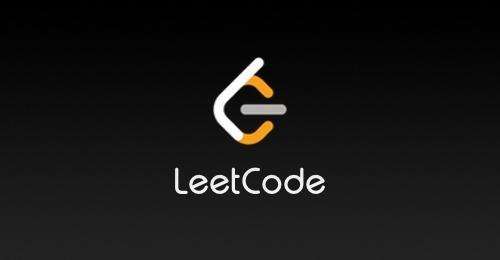
题目描述
给定一个非负索引 k,其中 k ≤ 33,返回杨辉三角的第 k 行。
在杨辉三角中,每个数是它左上方和右上方的数的和。
示例:
进阶:
你可以优化你的算法到 O(k) 空间复杂度吗?
解题思路
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| class Solution { public List<Integer> getRow(int rowIndex) { List<List<Integer>> res = new ArrayList<>(); List<Integer> rowList = null; int[][] arr = new int[rowIndex+1][rowIndex+1]; for(int i=0;i<=rowIndex;i++){ rowList = new ArrayList<>(); arr[i][0] = 1; rowList.add(arr[i][0]); for(int j=1;j<=i;j++){ arr[i][j] = arr[i-1][j-1] + arr[i-1][j]; rowList.add(arr[i][j]); } if(i == rowIndex){ return rowList; } res.add(rowList); } return res.get(res.size() - 1); } }
|
那么如何达到O(K)的空间复杂度呢?其实没必要用一个数组来存储所有的元素,可以用一个list来存放上一行的数据,见下面代码:
提交代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| import java.util.*; class Solution { public List<Integer> getRow(int rowIndex) { List<Integer> curr = null; List<Integer> pre = null; for(int i=0;i<=rowIndex;i++){ curr = new ArrayList<>(); for(int j=0;j<=i;j++){ if(j == 0 || j == i){ curr.add(1); }else{ curr.add(pre.get(j-1)+pre.get(j)); } } pre = curr; } return curr; } }
|
结语
杨辉三角作为一个经典题目,在大学学习编程的时候或许就遇到过这个问题,其实还有很多很多的优化方案,希望自己以后能够多扩展思路,不能为了做题而做题,因此,总有一天我会回来的,将这道题目优化到底。